Retrieving payables needs to be done asynchronously:
- first, request a snapshot to be created – this will create a new request and put it in a queue;
- then, poll for the status of this request in intervals of at least 60 seconds - your request is ready when status is "COMPLETE";
- the snapshot of payables will remain available in cache for 12 hours and can be retrieved again and again during this time.
This asynchronous process is required because payables are data-rich objects that carry a lot of pre-accounting information: accounts, tax codes, line items, analytical fields and more.
Here is a simplified diagram of the flow:
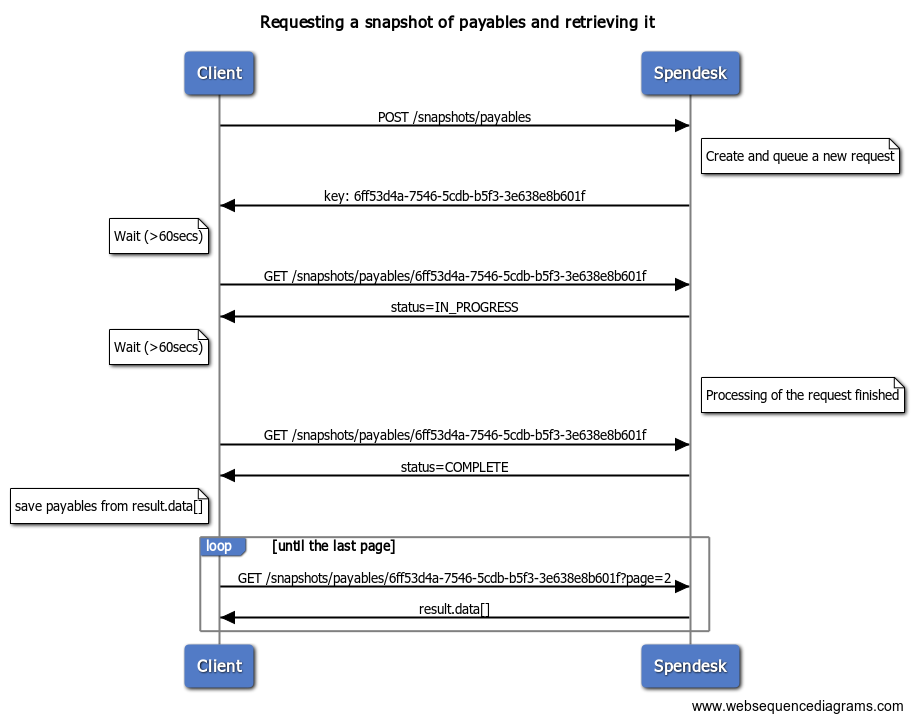
In more details, these are the steps to follow:
-
Request a "snapshot" of payables according to your desired criteria by using the Create a snapshot of payables API. This API will respond immediately with an ID - "key" - which will be the reference for your request.
//Request curl --request POST \ --url https://public-api.spendesk.com/v1/snapshots/payables \ --header 'accept: application/json' \ --header 'content-type: application/json' \ --header 'authorization: Bearer *****' \ --data ' { "sortBy": "payableDate", "sortOrder": "desc", "bookkeepingStatus": [ "exported", "exportedManually" ], "exportedAfter": "2024-01-01T00:00:00Z" } ' //Response 200 { "query": { "bookkeepingStatus": [ "exported", "exportedManually" ], "exportedAfter": "2024-01-01T00:00:00Z" "sortBy": "payableDate", "sortOrder": "desc", }, "key": "3fa85f64-5717-4562-b3fc-2c963f66afa6", "expireInSeconds": 43200, "remainingSeconds": 43200 }
-
Poll for the results every minute by using the Get a snapshot of payables API and "key" from the previous step.
curl --request GET \ --url https://public-api.spendesk.com/v1/snapshots/payables/3fa85f64-5717-4562-b3fc-2c963f66afa6 \ --header 'accept: application/json' \ --header 'authorization: Bearer *****'
-
Once you receive a response with status "COMPLETE" in the previous step, your data will be under result.data element. You may need to fetch multiple pages of results (see result.meta) using the same API.
-
In case you receive a response with status "ERROR" in step 2, stop polling and check your query.
Our recommendation is to retrieve payables once a day (at night) with the following query (setting updatedFrom to the day before):
curl --request POST \
--url https://public-api.spendesk.com/v1/snapshots/payables \
--header 'accept: application/json' \
--header 'authorization: Bearer *****' \
--header 'content-type: application/json' \
--data '
{
"updatedFrom": "2024-09-21",
"bookkeepingStatus": [
"prepared",
"exported",
"exportedManually",
"failedExport"
]
}
'