Before making any other calls to our API, you'll first need to authenticate. This can be done one of two ways:
Which method you use will depend on you use case, however consider the following guidance:
- For Spendesk customers wanting to build their own direct integration, the API Key flow is the simpler option
- For Spendesk partners wanting to build integrations that customers will use, the OAuth2 redirect flow is recommended.
Access Tokens
Your primary form of authenticating requests will be via an access token. These are temporary tokens you can retrieve via either of the above methods in order to validate subsequent requests to our other endpoints.
Access tokens are valid for 60 minutes, i.e. 3600 seconds, at which point any requests using them will return a
401
error and a new token will need to be requested.⚠️ As with all things related to security, tokens should be handled securely. Don't store access tokens in persistent storage and before every call check if the token is still valid. If it's not, request a new one.
You can see how the access tokens are used in this quickstart example.
To find out more detail on how to first get your access token, read on.
API Keys
Using the API Key flow is the simpler of the two options and requires a single request for the access token to /auth/token.
You can manage your API keys directly by logging into Spendesk and navigating to Settings -> Integrations
.
API keys can be valid for up to a maximum of 1 year.
This request uses your API Key configuration, you must provide your id and secret in the format id:secret
then base64 encode it. You'll end up with something like:
- plain:
my_id:my_secret
- encoded:
bXlfaWQ6bXlfc2VjcmV0
Then you're ready to make a call to authenticate at /auth/token
using that encoded value in the Authorization
header. Don't forget the word Basic
in front of the encoded value, so that your HTTP header looks like this: Authorization: Basic bXlfaWQ6bXlfc2VjcmV0
To get a new token, for example when one has expired, simply make another call to the /auth/token
endpoint using the id and secret.
Our quickstart guide covers this flow end-to-end, so we recommend following that to get a feel for this.
OAuth2
We use a common OAuth2 redirect flow for when client credentials are required to authenticate on behalf of a customer. This follows these general steps:
- Your system first makes a call to our
/oauth2/authorize
endpoint using your client idredirect_uri
should start withhttps
(with ans
at the end!) and should be hosted on the domain provided to Spendesk when creating your new partner record (often onlocalhost
for development purposes)- use this tool to easily generate
code_challenge
to get you started
- We respond with HTTP code 302 Redirect with a URL to the Spendesk frontend which your system should redirect to
- On Spendesk, the customer can login and approve the OAuth connection between our systems
- Once successful, Spendesk redirects back to your frontend with a connection
code
in the URL - Your system makes a final call to our
/oauth2/token/create
endpoint using the connectioncode
andcode_verifier
generated in the first step above - If this is successful, we send back both an access token as well as a refresh token
- Use the access token in any subsequent API calls for this customer, for example to
/settlements
- Use the refresh token to request new access tokens using the
/oauth2/token/refresh
endpoint
Access tokens via OAuth2 are scoped to the specific organisation that approved the connection.
When the access token is expired, instead of starting the flow again, the /oauth2/token/refresh
endpoint should be used to 'refresh' the connection and generate a new token. You can either:
- Wait until you see a
401
to request a new token via the/refresh
- Proactively request new tokens before the 1 hour expiry time. Store the new refresh token in your database.
Refresh tokens expire in 30 days. We recommend you store them in your database along with your internal customer ID and expiration date. If there is a possibility that no API calls are made for 30 days for a given customer, then request a new refresh token before the current one expires in order to keep the connection alive. If you are unable to get a new refresh token (with a 401
or 403
error codes), remove the token from your database and "disconnect" the customer.
OAuth2 for customers with several entities
Many customers have more than one wallet in Spendesk. Typically they would want to connect each wallet. In such case each OAuth2 connection is independent, we don't provide a way to connect all companies belonging to the same parent organization at once. However the connction process starts from the same partner account, that's how you know they belong to the same customer of yours.
When such customer arrives on Spendesk to authorize an OAuth2 connection, they have to pick the company they are connecting in the dropdown (in the screenshot there is just one, but for multi-entity customers there will be several). They will need to repeat this process for every company/wallet they have with us, each time selecting a different company in the dropdown.
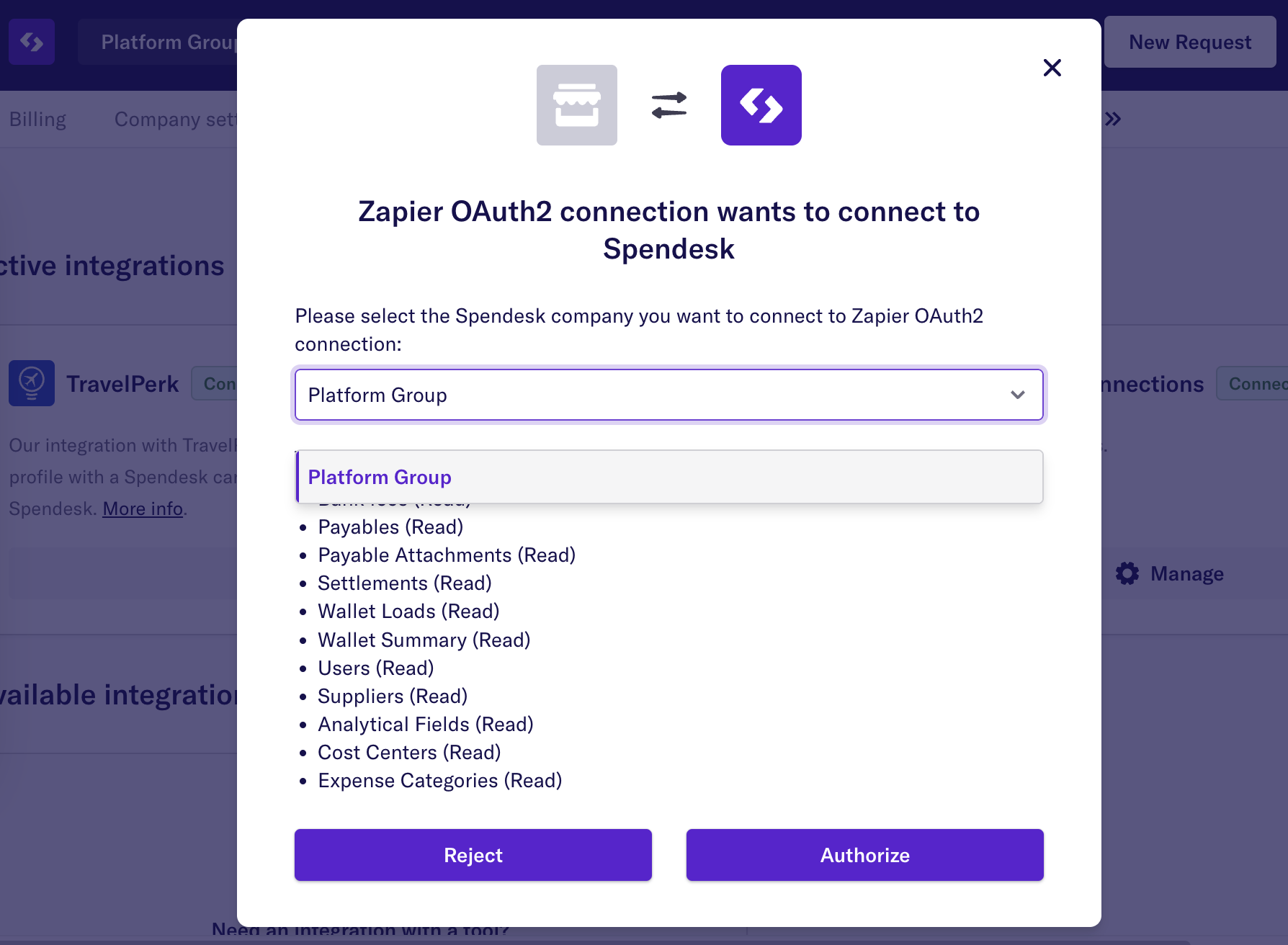
Once you get an access token for each new connection, you can either call Get wallet summary endpoint (with no parameters) to get the name of the wallet (company), or you can decode the access_token on jwt.io to get the ID of the wallet (company).